Subscriptions
Introduction
Lukapay subscriptions allow you to easily accept recurring credit and debit card payments. When using this library, Lukapay affiliates a customer’s data through a JavaScript library without needing PCI compliance, as the buyer’s payment data is tokenized and sent directly to payment processors' servers.
Once the customer’s credit card is affiliated, the company can make payments through an API without needing the customer’s prior approval. The system allows listing a subscriber’s cards and deleting them if payments with that card are no longer desired.
Requirements
To affiliate the subscriber and their payment methods, it is necessary to integrate the Lukapay JavaScript library.
Afterward, through our REST API, you can make recurring payments, list, and delete a customer’s credit cards.
Available Methods
The subscription process is divided into two parts:
Create subscriber token: Credit/Debit card affiliation and storage in the vault via Lukapay JS.
Checkout: Create a transaction for an existing buyer via REST API:
- Authentication
- Retrieve the customer's card list
- Pay with a vault-stored credit card
- Delete a credit card from the vault.
Create Subscriber Token
Below are the specifications for implementing the Lukapay JavaScript library, which will be used for customer affiliation and tokenization of their payment methods.
Payment Methods
Name | Currency | Key | Environment |
---|---|---|---|
Credit/Debit Card | USD | tdc_bs | Production |
Credit/Debit Card | CLP | tbk_oneclick | Testing |
Luka JS Implementation
See Luka JS Library Documentation.
Register Card
Depending on the selected currency, the user must complete the registration form by entering credit/debit card information.
Registration Form for USD
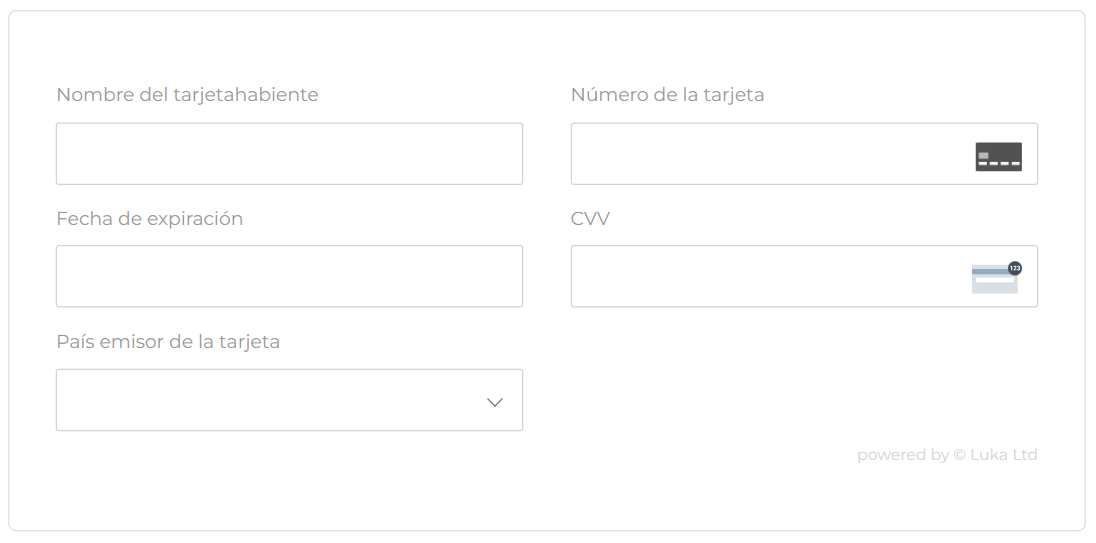
Checkout
This method is used to generate a recurring charge for the registered buyer from the merchant's application, which controls the payment flow from end to end.
Necessary steps for this process:
- Generate a token for application authentication.
- Collect and send the buyer's payment information via REST API.
- Retrieve the response object
LukaPaymentResult
. - Complete the transaction within the merchant's application.
The URL is specified in Environments.
Authentication is performed via a token (JWT) returned by the login method using the credentials provided by Lukapay. See Documentation.
Retrieve Customer Card List
This method allows you to get the list of cards that the customer has registered in the vault. This step is essential to specify which credit card will be used to make payments in US dollars (USD).
To retrieve the customer's cards, you must provide the LukapayId
value, which is returned when creating the card token, and the merchant must associate it with the user in their own database.
Request
GET {URL}/api/v1/tarjetacredito/servicio/{LukapayId}
Headers
Content-Type: application/json
Authorization: Bearer {token}
Response
Body
The response returns a list of objects containing the following credit/debit card information:
Field | Description | Type |
---|---|---|
Id | Unique identifier of the card in the Lukapay database | Number |
UltimosCuatroDigitos | Last four digits of the card | String |
SubTipoTarjeta | Indicates whether the card is credit or debit | String |
TipoTarjeta | Card type. Example: VISA, MASTER | String |
CategoriaTarjeta | Indicates whether the card is commercial or personal | String |
Bin | Bank Identification Number (BIN). These are the first 4 to 6 digits of the credit card number | String |
FechaVencimiento | Expiration date of the card in MM/YYYY format | String |
Pais | ISO code of the country where the card was issued | String |
EstaBoveda | Indicates if the card is associated with the user's vault | Boolean |
Direccion | Object with the billing address of the card | Object |
Descripcion | Name or description provided by the user to easily identify the card | String |
Example Usage
Request
GET {URL}/api/v1/tarjetacredito/servicio/560356a272d9
Response Body
[
{
"Id": 1,
"UltimosCuatroDigitos": "1111",
"SubTipoTarjeta": "CREDIT",
"TipoTarjeta": "VISA",
"CategoriaTarjeta": "CONSUMER",
"Bin": "111111",
"FechaVencimiento": "03/2023",
"Pais": "US",
"EstaBoveda": true,
"Direccion": {
"Id": 0,
"Direccion": "Calle 1",
"Ciudad": "Guatire",
"Estado": "Miranda",
"CodigoPostal": "1111"
},
"Descripcion": ""
}
]
Pay with a Credit Card from the Vault
This function allows you to make a payment request using the credit/debit card information previously registered by the user.
Pay in USD
This method requires the credit/debit card information obtained from the "Get Customer Card List" flow to proceed with the payment.
Request
POST {URL}/api/v1/transaccion
Headers
Content-Type: application/json
Authorization: Bearer {token}
Body
Name | Description | Type | Required |
---|---|---|---|
EmailTarjetaHabiente | User's email | String | Yes |
IdCanal | Channel ID through which the service is being consumed. Default value: 2 | Number | No |
IdTraza | Transaction ID in the merchant's system. This field will be used later to check the status of the transaction. | String | Yes |
Moneda | ISO currency code. Example: USD, CLP | String | No |
Monto | Amount of the transaction or payment | Number | Yes |
Referencia | Payment identifier visible to the customer. Example: invoice number, contract ID, etc. | String | No |
TarjetaCredito | Information of the card to be used for the payment. Obtained from the "Get Customer Card List" method. | Object | Yes |
TarjetaHabiente | Information about the paying user | Object | Yes |
Properties of the Objects
TarjetaCredito
Name | Description | Type |
---|---|---|
Id | Card ID in the Lukapay database (Required) | Number |
UltimosCuatroDigitos | Last four digits of the card | String |
SubTipoTarjeta | Indicates whether the card is credit or debit | String |
TipoTarjeta | Indicates the type of credit card. Example: VISA, MASTER | String |
CategoriaTarjeta | Indicates whether the card is commercial or personal | String |
Bin | Bank Identification Number (BIN). These are the first 4 to 6 digits of the credit card number | String |
FechaVencimiento | Expiration date of the card in MM/YYYY format | String |
Pais | ISO code of the card's issuing country | String |
TarjetaHabiente
Name | Description | Type | Required |
---|---|---|---|
Apellido | User's last name | String | No |
Nombre | User's first name | String | No |
NumeroIdentificacionPersonal | User's ID number | String | No |
NumeroTelefono | User's phone number | String | No |
LukapayId | User's registration ID in the card vault (UUID) | String | Yes |
Response
Name | Description | Type |
---|---|---|
Canal | Indicates the channel used to process the payment in Lukapay | Number |
CargosAdicionales | Object containing additional charges | Object |
Cuotas | Object containing information about installment payments | Object |
Descripción | Contains the payment application response, such as transaction status and any additional information | String |
Exitoso | Indicates whether the transaction was successful. Used to validate the response | Boolean |
FechaOperacion | Date the transaction took place | String |
InfoProceso | Object containing more detailed status information of the transaction | Object |
InfoTarjeta | Object with information about the credit card used for the payment. Applies only for card payments | Object |
InfoUsuarioPagador | Object that returns basic information about the paying user | Object |
MedioDePago | Indicates the payment method used | String |
MerchantId | Merchant transaction reference used to apply the payment | String |
Moneda | Currency code used for the payment | String |
Monto | Indicates the amount of the payment made | Number |
MontoOriginal | Object containing information on the original amount. Used when currency conversion is required | Object |
MontoUsd | Payment amount in US dollars. Used when MontoOriginal is specified | Number |
TarjetaHabiente | Object containing information about the payer (if specified) | Object |
TransaccionId | Lukapay transaction reference | Number |
TransaccionMerchantId | Merchant transaction reference used to apply the payment | Number |
TrazaId | Internal merchant identifier. If not provided, a random code is generated | String |
Example Usage
{
"Monto":100,
"TarjetaHabiente": {
"Nombre":"Jhon",
"Apellido":"Doe",
"LukapayId":"560356a272d9"
},
"TarjetaCredito": {
"Id": 1,
"UltimosCuatroDigitos": "1111",
"SubTipoTarjeta": "CREDIT",
"TipoTarjeta": "VISA",
"CategoriaTarjeta": "CONSUMER",
"Bin": "111111",
"FechaVencimiento": "03/2023",
"Pais": "US",
},
"IdTraza":"1234567890",
"Moneda":"USD",
"EmailTarjetaHabiente":"usuario@mail.com",
"IdCanal":2,
"Referencia":"000265700237"
}
{
"Monto": 100.0,
"MontoUsd": 100.0,
"InfoProceso": {
"EstatusProcesamiento": "success",
"CodigoRespuestaCvv": "N/D"
},
"TarjetaHabiente": {
"Nombre":"Jhon",
"Apellido":"Doe",
“LukapayId”:”560356a272d9”
"NumeroIdentificacionPersonal": null,
"NumeroTelefono": null,
"LukapayId": "560356a272d9"
},
"InfoUsuarioPagador": {
"Nombre": "Jhon",
"Apellido": "Doe",
"NumeroIdentidad": null,
"NumeroTelefono": null,
"Email": "usuario@mail.com"
},
"Moneda": "USD",
"InfoTarjeta": {
"Id": 1,
"UltimosCuatroDigitos": "1111",
"SubTipoTarjeta": "CREDIT",
"TipoTarjeta": "VISA",
"CategoriaTarjeta": "CONSUMER",
"Bin": "111111",
"FechaVencimiento": "03/2023",
"Pais": "US",
},
"TransaccionId": 102143,
"TransaccionMerchantId": 1108334200,
"Descripcion": "success",
"TrazaId": "1234567890",
"Exitoso": true,
"Canal": "Api",
"MedioDePago": "Débito",
"MontoOriginal": null,
"MerchantId": null,
"FechaOperacion": null,
"CargosAdicionales": null,
"Cuotas": null
}
Pay with CLP
To process a payment in Chilean pesos (CLP) using a previously registered card, you only need to provide the LukapayId along with the transaction details. Below are the method specifications.
Request
POST {URL}/api/v1/transaccion/transbank.authorize
Headers
Content-Type: application/json
Authorization: Bearer {token}
Request Body
Name | Description | Type | Required |
---|---|---|---|
User's email address | String | Yes | |
TrazaId | Identifier of the transaction in the merchant's system. This field will be used later to check the status of a transaction. | String | Yes |
Moneda | ISO currency code. Example: CLP | String | Yes |
Monto | Amount of the transaction or payment | Number | Yes |
*Referencia | Identifier of the payment for the client. Example: invoice number, contract ID, etc. | String | No |
LukapayId | Identification of the user record in the vault of cards. | String | Yes |
Response
Field Name | Description | Type |
---|---|---|
Canal | Channel used to process the payment | Number |
CargosAdicionales | Additional charges details | Object |
Cuotas | Information about payment installments | Object |
Descripción | Response providing the transaction status and any additional information | String |
Exitoso | Indicates if the transaction was successful | Boolean |
FechaOperacion | Date and time of the transaction | String |
InfoProceso | Detailed information about the transaction status | Object |
InfoTarjeta | Credit card details used for payment (if applicable) | Object |
InfoUsuarioPagador | Information about the customer making the payment | Object |
MedioDePago | Payment method used | String |
MerchantId | Merchant's transaction identifier | String |
Moneda | Currency used for the payment | String |
Monto | Amount paid | Number |
MontoOriginal | Information about the original amount (if currency conversion applies) | Object |
MontoUsd | Value of the amount in U.S. dollars | Number |
TarjetaHabiente | Payer's details if a card is used | Object |
TransaccionId | Transaction identifier in Lukapay | Number |
TransaccionMerchantId | Transaction identifier from the merchant | Number |
TrazaId | Unique identifier for the transaction in the merchant's database | String |
For more details on the response objects, see Transaction Response.
Example
Request body
{
"Moneda": "CLP",
"Monto": 100,
"TrazaId": "1234567890",
"LukapayId": "560356a272d9",
"Email": "example@mail.com",
"Referencia": ""
}
Response body
{
"Monto": 100.0,
"MontoUsd": 0.0,
"InfoProceso": {
"EstatusProcesamiento": "success",
"CodigoRespuestaCvv": null
},
"TarjetaHabiente": {
"Nombre": "John",
"Apellido": "Doe",
"NumeroIdentificacionPersonal": "",
"NumeroTelefono": null,
"LukapayId": "6353a328-ef92-454d-826b-f59a01ca62d3"
},
"InfoUsuarioPagador": {
"Nombre": "John",
"Apellido": "Doe",
"NumeroIdentidad": null,
"NumeroTelefono": null,
"Email": "example@mail.com"
},
"Moneda": "CLP",
"InfoTarjeta": null,
"TransaccionId": 102144,
"TransaccionMerchantId": 1108334200,
"Descripcion": "transacción exitosa",
"TrazaId": "1234567890",
"Exitoso": true,
"Canal": "Api",
"MedioDePago": "Transbank",
"MontoOriginal": null,
"MerchantId": null,
"FechaOperacion": null,
"CargosAdicionales": null,
"Cuotas": null
}
Verify the Status of a Transaction
Delete a Credit Card from the Vault
This method allows you to remove a credit card from the vault. You need to provide the card ID to be deleted and the LukapayId of the user.
Request
- For USD:
DELETE {URL}/api/v1/tarjetacredito/{idTarjeta}/user/{LukapayId}
- For CLP:
DELETE {URL}/api/v1/transaccion/transbank.unsubscribe/{LukapayId}
Headers
Content-Type: application/json
Authorization: Bearer {token}
Response
The method returns a status code of 202 (Accepted) if the operation is successful; otherwise, it returns an error code.
Errors Code
Code | Message | Translation Message |
---|---|---|
400 | la moneda no está soportada | the currency is not supported |
400 | el correo electrónico es obligatorio | the email address is required |
400 | la tarjeta ya se encuentra registrada | the card is already registered |
400 | no se consiguió la tarjeta de crédito | the credit card could not be found |
400 | ocurrió un error verificando la información de la tarjeta de crédito | an error occurred verifying the credit card information |
400 | identificador de tarjeta inválido | invalid card identifier |
400 | identificador de usuario inválido | invalid user identifier |
401 | acceso no autorizado | unauthorized access |
401 | usuario no registrado | user not registered |
401 | no se consiguieron parámetros de consulta válidos | valid query parameters not found |
402 | monto incorrecto | incorrect amount |
404 | no se encontró la tarjeta de crédito | credit card not found |
409 | ocurrió un error procesando la transacción | an error occurred processing the transaction |
500 | ocurrió un error inesperado | an unexpected error occurred |
500 | monto menor o igual a cero | amount less than or equal to zero |
500 | no se puede procesar la validación de la tarjeta porque el usuario del servicio no está registrado | cannot process card validation because the service user is not registered |